
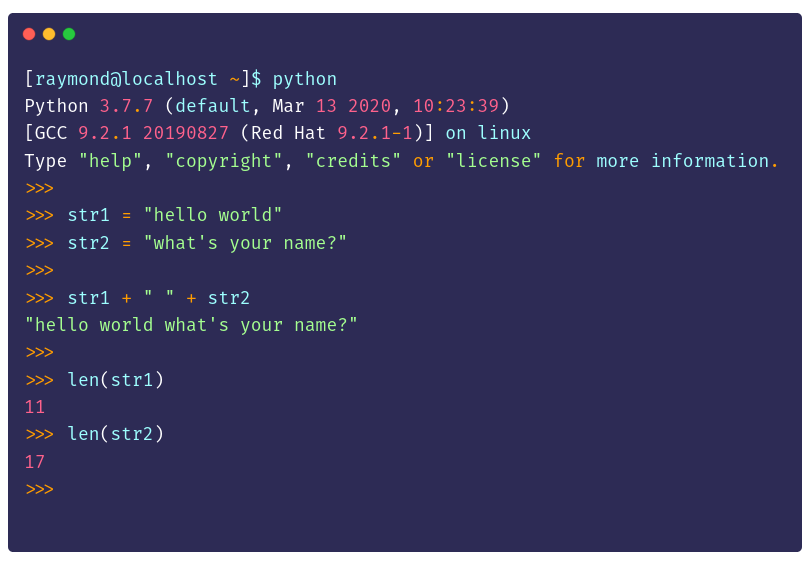
Let's see the table of contents of the tutorial. Consequently, in this tutorial, we are going to see the remaining data types of Python. However, there are more Python Literal and Data Types. You can see that using a simple Boolean check, how easy it becomes to build a function that returns whether a number is even or odd.We have seen the int and float data types in the previous tutorial.
#Python convert string to boolean expression code
Let’s code out a function that uses Boolean to determine whether a number is odd or even.ĭef func(value): return (bool(value%2=0)) if(func(9)): print("It\'s Even") else: print("It\'s Odd") Whether it’s an if-else condition, a simple function, or even a for-loop, Boolean is often used either directly or in disguise. The use of Boolean in Python is inevitable. The method endswith() returns True if the string ends with the letter mentioned in the argument. The method istitle() returns True if the string has all its words starting with an upper-case letter. The method isalnum() returns whether or not the string is alpha-numeric. > my_string = "Welcome To Simplilearn" > my_string.isalnum() > my_string.istitle() > my_string.endswith("n") Several in-built functions return a Boolean in Python. In fact, many in-built functions in Python return their output in the form of Boolean values.ĭef simplilearn(): return True if simplilearn() = True: print("I use simplilearn") else: print("I don't use simplilearn") You can also create functions in Python that return Boolean Values. Using Functions that Return Boolean in Python > True or True > False or True > True or False > False or False Hence, it also uses short-circuit evaluation. If the first argument is True, then it’s always true. The Or operator returns False only when both the inputs to the operator are False, else it always returns True. > True and True > False and True > True and False > False and False In such cases, knowing only one input is enough, and hence, the other input is not evaluated. Please note that if the value of A is False, then the value of B doesn’t matter. It takes two input arguments and evaluates to True only if both the arguments are True. The truth table for Not operator is mentioned below.
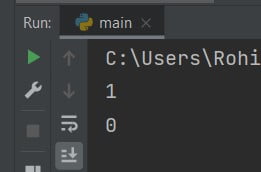
For example, if the argument is True, it returns False and vice-versa. The Not operator takes only one argument and it just returns the opposite result. Broadly, we have three boolean operators in Python that are most frequently used. > bool (846.23 > 846.21) > bool (0=1)īoolean operators take Boolean values as inputs and in return, they generate a Boolean result. The method will determine if the expression evaluates to True or False. You can also use the bool method with expressions made up of comparison operators. In the following example, we have cast an integer into boolean type. You can use the bool method to cast a variable into Boolean datatype. Usually, empty values such as empty strings, zero values, and None, evaluate to False. Moreover, apart from the empty ones, all the sets, lists, tuples, and dictionaries also evaluate to True. In fact, except for empty strings, all the strings evaluate to True. Mostly, if any value has some type of content in it, it is finally evaluated to True when we use the bool() method on it. > A = 1.123 > bool(A) > A = 23 > B = 23.01 > bool(A=B)Įvaluation of Boolean Expressions in Python It returns True if the value of x is True or it evaluates to True, else it returns False. However, passing a parameter to the bool() method is optional, and if not passed one, it simply returns False. It takes one parameter on which you want to apply the procedure. The bool() method in Python returns a boolean value and can be used to cast a variable to the type Boolean. However, it’s not a good practice to do so.īool = "Welcome to Simplilearn" print(bool) This means that you can assign a variable with the name bool.
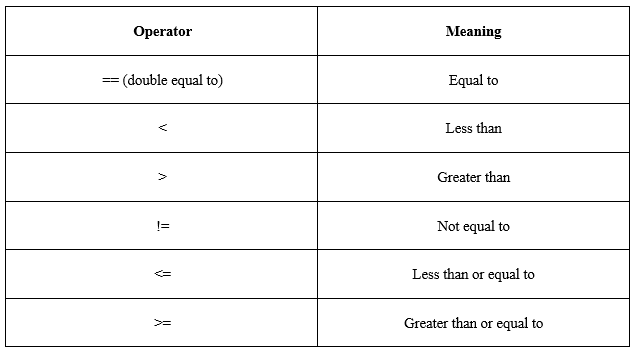
Note that the type function is built-in in Python and you don’t have to import it separately.Īlso, the word bool is not a keyword in Python. You can check the type of the variable by using the built-in type function in Python. If you want to define a boolean in Python, you can simply assign a True or False value or even an expression that ultimately evaluates to one of these values.
